Issue
You would like to delete multiple jobs simultaneously in folders, delete multiple jobs of a certain type or block certain job types from being created on your controller.
Environment
-
CloudBees CI (CloudBees Core) on modern cloud platforms - Managed controller
-
CloudBees CI (CloudBees Core) on modern cloud platforms - Operations Center
-
CloudBees CI (CloudBees Core) on traditional platforms - Client controller
-
CloudBees CI (CloudBees Core) on traditional platforms - Operations Center
-
CloudBees Jenkins Enterprise
-
CloudBees Jenkins Enterprise - Managed controller
-
CloudBees Jenkins Enterprise - Operations center
Resolution
1. If you would like to block certain Job types from being created in Jenkins the recommended way is to use Folders from the Folders Plugin
. Using these folders you should configure Users into Groups and give these groups Roles that allow for them to only create and run jobs in specific folders meant for these Groups.
Then you may select Configure
on these folders, go to the bottom of that page where you see a checkbox, Restrict the kind of children in this folder
select this checkbox and you may now select the specific kinds of jobs you would like to allow within this folder.
The image example below shows how to restrict a Group’s folder to not being able to create a FreeStyle Job:
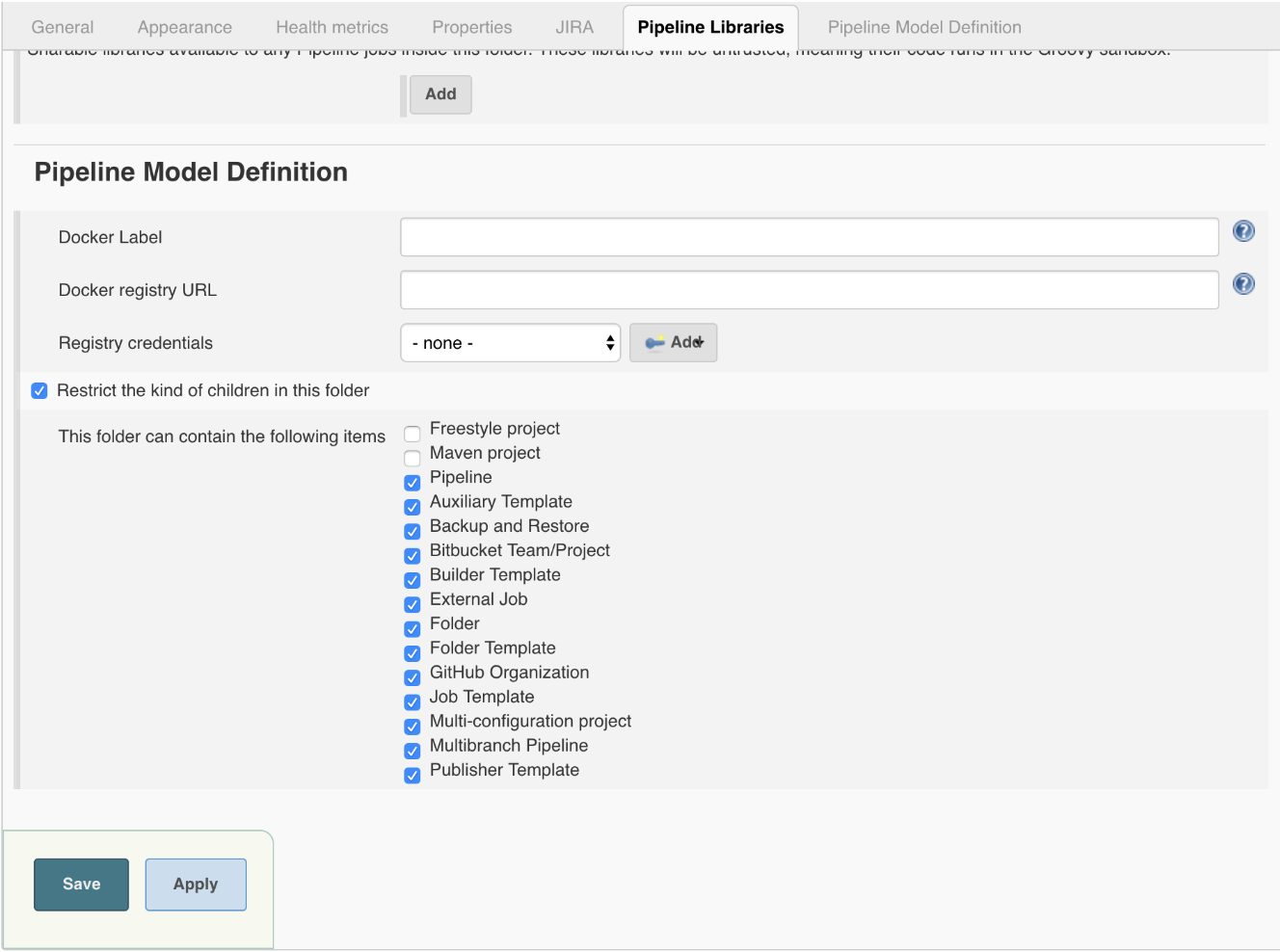
NOTE: There is no undo for any Groovy Scripts once they have been ran and the jobs are deleted. It is always heavily recommended to take a backup before deleting anything or running any Groovy Scripts on your Jenkins instance.
2. To delete a set of specific Jobs by name you can run the following Groovy Script from the Script Console ($JENKINS_URL/script
):
import hudson.model.* jobsToDelete = ["aFolder/aJobInFolder","topLevelJob"] deleteChildren(Hudson.instance.items) def deleteChildren(items) { items.each { item -> if (item.class.canonicalName != 'com.cloudbees.hudson.plugins.folder.Folder') { if (jobsToDelete.contains(item.fullName)) { println(item.fullName) //item.delete() } } else { deleteChildren(((com.cloudbees.hudson.plugins.folder.Folder) item).getItems()) } } }
Replace jobsToDelete
values with your job names. Note that for jobs in folders use /
separator with folder names, i.e. aFolder/aJobInFolder
.
Running with //item.delete()
allows you to see a list of jobs that would be deleted without actually deleting any (dry run). Once you are happy with the job list uncomment item.delete()
, i.e. //item.delete()
-> item.delete()
.
3. If you would like to delete ALL MultiBranch Jobs then you may use the below Groovy Script from the Script Console
import jenkins.model.Jenkins import hudson.model.Job import org.jenkinsci.plugins.workflow.multibranch.WorkflowMultiBranchProject //Input: //If dryRun is true, will print list of jobs that would be included in the deletion def dryRun = true Jenkins.get().getAllItems(WorkflowMultiBranchProject.class).each { WorkflowMultiBranchProject job -> if (dryRun) { println "${job.fullName} will be removed." } else { job.doDisable() job.delete() println "${job.fullName} has been removed." } } return
Running with dryRun=true
allows you to see a list of jobs that would be deleted without actually deleting any. Once you are happy with the job list, run with dryRun=false
WARNING Please note that this will delete ALL MultiBranch jobs in your instance, at the root and all folders and nested folders.
4. If you would like to delete ALL jobs of FreeStyle or Pipeline (WARNING This INCLUDES Pipeline Branches in MultiBranchPipeline Folders) type then you may use the below Groovy Script from the Script Console ($JENKINS_URL/script
):
import jenkins.model.Jenkins import hudson.model.Job import hudson.model.FreeStyleProject import org.jenkinsci.plugins.workflow.job.WorkflowJob //Inputs: //If dryRun is true, will print list of jobs that would be included in the deletion def dryRun = true //Copy one of these Job Types available for deletion: FreeStyleProject, WorkflowJob def jobDeleteType = JOB_TYPE //Script: Jenkins.instance.getAllItems(Job.class) .findAll { Job job -> (job in jobDeleteType) } .each { Job job -> def jobName = "${jobDeleteType} Job Name: ${job.fullName}" if (dryRun) { println "${jobName} will be removed." } else { job.delete() println "${jobName} has been removed." } } return
WARNING Please note that this will delete ALL jobs in your instance of this specific type, throughout the root location and all folders and nested folders.
You may use either FreeStyleProject
within the jobDeleteType
variable to delete all FreeStyle jobs or use WorkflowJob
to delete all Pipeline Projects.
Running with dryRun=true
allows you to see a list of jobs that would be deleted without actually deleting any. Once you are happy with the job list, run with dryRun=false