The following examples show how to use the REST API as well as highlight the benefits of deploying it in your environment.
Create a simple flag
The following code can be used to create a simple flag default.followingView
that will return true
for all users:
var request = require("request"); var options = { method: 'PUT', url: 'https://x-api.rollout.io/public-api/applications/5990c4a1eae09726fa0d6041/flags', body: JSON.stringify({ "name": "default.followingView" }), headers: { authorization: 'Bearer XXXX-XXXX-XXXX-XXXX-XXXXX', 'content-type': 'application/json' } }; request(options, function (error, response, body) { if (error) throw new Error(error); console.log(body); });
You can also create a flag using the UI. For instructions refer to Creating feature flags.
Any flags that you create, whether created in the UI or in code, are listed on Flags overview and on the environment’s overview page.
Create a flag with a configuration
The following code can be used to create a flag default.followingView
that will return true
for all users in the Production environment. If following along this will update the flag from the previous step, but only in the Production environment:
var request = require("request"); var options = { method: 'PUT', url: 'https://x-api.rollout.io/public-api/applications/5990c4a1eae09726fa0d6041/Production/flags/default.followingView', body: JSON.stringify({ "name": "following view", "value": true }), headers: { authorization: 'Bearer XXXX-XXXX-XXXX-XXXX-XXXXX', 'content-type': 'application/json' } }; request(options, function (error, response, body) { if (error) throw new Error(error); console.log(body); });
The resulting flag audience looks like this:
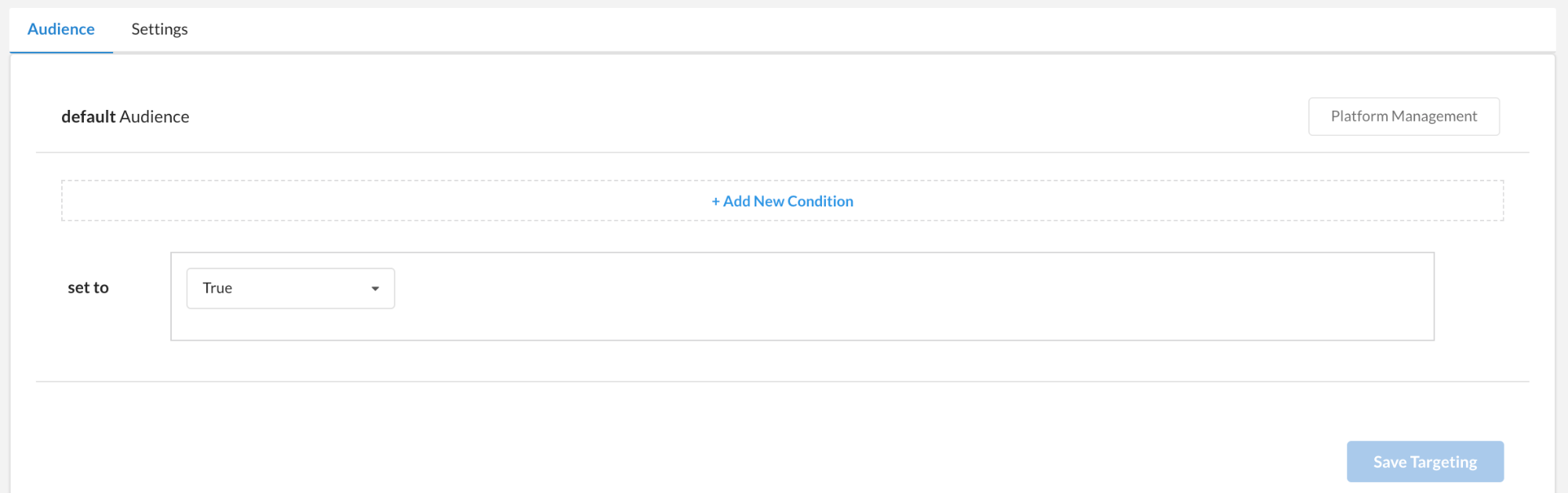
The flag’s Settings look like this:
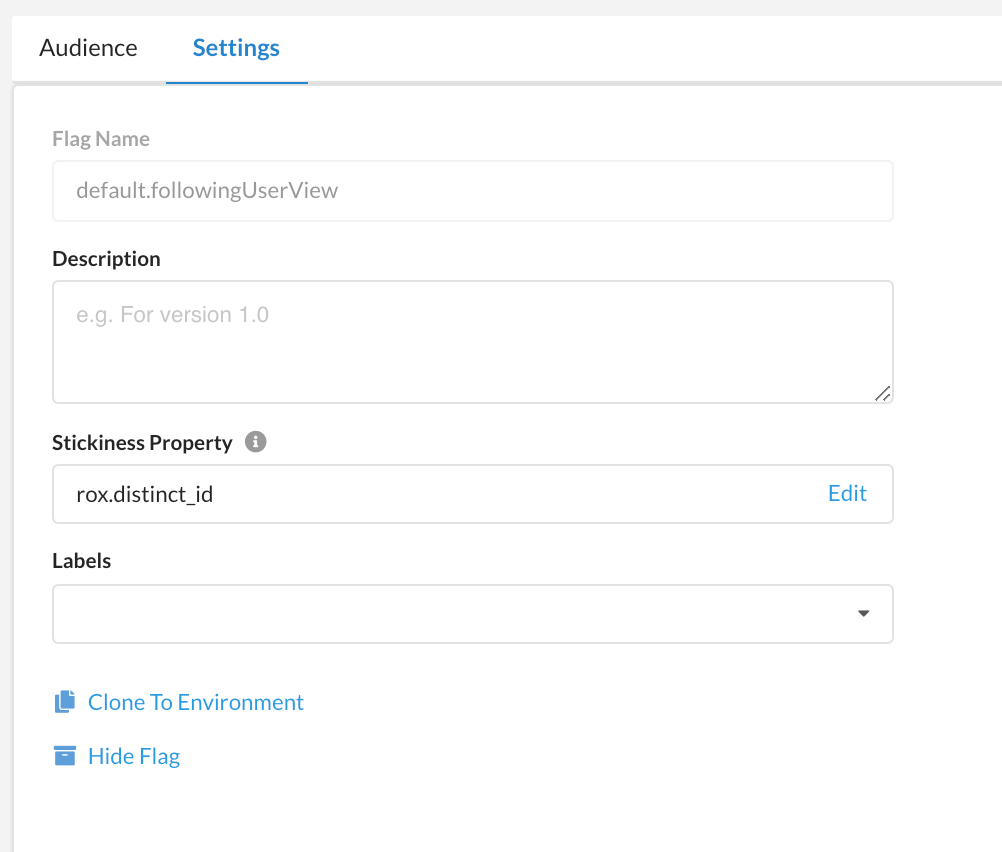
Create a flag with property matching
The following code will create a flag that is opened for users with email bob@example.com.
This will override existing flag default.followingView
it if already exists for the production environment.
var request = require("request"); var options = { method: 'PUT', url: 'https://x-api.rollout.io/public-api/applications/5990c4a1eae09726fa0d6041/Production/flags/default.followingView', body: JSON.stringify({ "name": "following view", "conditions": [ { "property": { "name": "email", "operator": "in-array", "operand": [ "bob@example.com" ] }, "value": true } ], "value": false }), headers: { authorization: 'Bearer XXXXX-XXX-XXXX-XXXX-XXXXXX', 'content-type': 'application/json' } }; request(options, function (error, response, body) { if (error) throw new Error(error); console.log(body); });
The result flag audience looks like this:
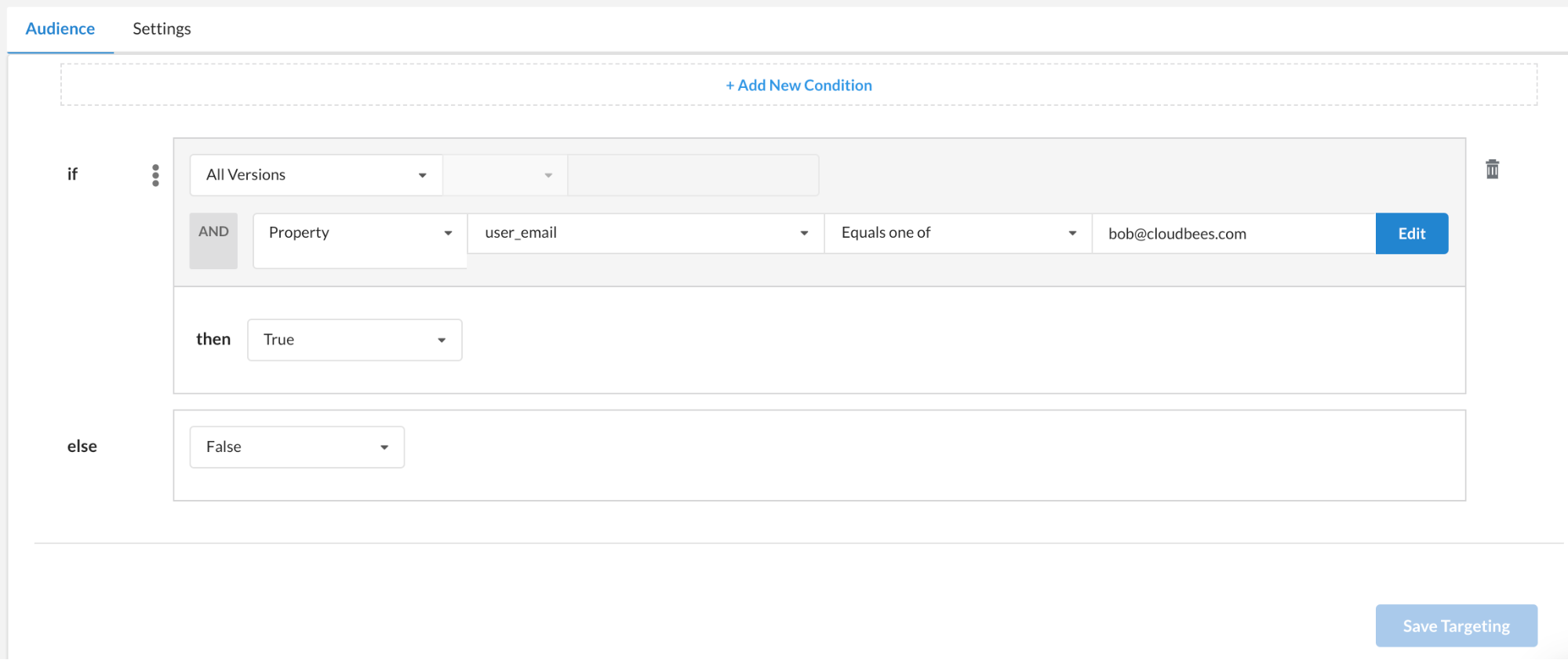
Add value to property matching
In this example, you can modify an existing flag by using the following code to get the flag value.
var request = require("request"); var options = { method: 'GET', url: 'https://x-api.rollout.io/public-api/applications/5990c4a1eae09726fa0d6041/Production/flags/default.followingView', headers: { authorization: 'Bearer XXXXX-XXXX-XXXX-XXXX-XXXXXXX', 'content-type': 'application/json' } }; request(options, function (error, response, body) { if (error) throw new Error(error); console.log(body); });
The resulting JSON looks like this:
{ "name": "default.followingView", "availableValues": [ true, false ], "description": "", "created": "Fri Mar 05 2021 14:45:13 GMT+0000 (Greenwich Mean Time)", "updated": "Fri Mar 05 2021 14:45:13 GMT+0000 (Greenwich Mean Time)", "enabled": true, "labels": [], "stickinessProperty": "rox.distinct_id", "platforms": [], "conditions": [ { "property": { "name": "email", "operator": "in-array", "operand": [ "bob@example.com" ] }, "value": true } ], "value": false }
Next, add dylan@example.com
to the emails who get the value true
using the PATCH
command:
var request = require("request"); var options = { method: 'PATCH', url: 'https://x-api.rollout.io/public-api/applications5990c4a1eae09726fa0d6041/Production/flags/default.followingView', body: JSON.stringify([{ path: "/conditions/0/property/operand/0", op:"add", value:"dylan@example.com" }]), headers: { authorization: 'Bearer XXXXX-XXXX-XXXX-XXXX-XXXXXXX', 'content-type': 'application/json' } }; request(options, function (error, response, body) { if (error) throw new Error(error); console.log(body); });
If you query the flag again, you can see dylan@example.com
:
{ "name": "default.followingView", "availableValues": [ true, false ], "description": "", "created": "Fri Mar 05 2021 14:45:13 GMT+0000 (Greenwich Mean Time)", "updated": "Fri Mar 05 2021 14:45:13 GMT+0000 (Greenwich Mean Time)", "enabled": true, "labels": [], "stickinessProperty": "rox.distinct_id", "platforms": [], "conditions": [ { "property": { "name": "email", "operator": "in-array", "operand": [ "bob@example.com", "dylan@example.com" ] }, "value": true } ], "value": false }
Delete a flag
The code block below demonstrates how to delete a flag.
var request = require("request"); var options = { method: 'DELETE', url: 'https://x-api.rollout.io/public-api/applications/5990c4a1eae09726fa0d6041/flags/default.followingView', headers: { authorization: 'Bearer XXXX-XXXX-XXXX-XXXX-XXXXX', 'content-type': 'application/json' } }; request(options, function (error, response, body) { if (error) throw new Error(error); console.log(body); });
Flags can also be deleted using the dashboard. Refer to Deleting feature flags for instructions.
Remove write permissions from a specific environment
The following code demonstrates how you can remove write permissions from a specific application’s environment using the PATCH
command.
#!/bin/bash token=<put token> email=<put email> app_id=<put id> environment_name=<put env name> encoded_email=$( echo $email | sed 's/@/%40/g') #simple email (url) encoder curl --request PATCH \ --url https://x-api.rollout.io/public-api/users/$encoded_email \ --header "authorization: Bearer $token" \ --header "content-type: application/json" \ --data "[{\"op\":\"remove\",\"path\":\"/applications/$app_id/environments/$environment_name\",\"value\":{}}]"