A target group is a set of users having the same properties or attributes, such as email domain, language, geolocation, device, or software version.
Create an unlimited number of target groups in the CloudBees platform. Each target group configuration can have nested conditions for complex logic and unlimited attributes, allowing you to finely tune a given audience for a feature flag.
Multiple flags can all have the same targeting, and updating a single target group definition applies to all flags that use the target group in their flag configurations.
Set up a target group for internal testing only, for example, and use it across multiple flags to test new features. Once internal testing is complete, modify the target group to roll out the new features to external users.
Create target groups using custom or built-in properties defined in the code. All target groups and property configurations are scoped to the application where they are defined. They are not shared across applications.
Learn more about defining properties in the SDK references, and using properties and target groups to configure flags.
Create custom properties in either the UI or in code.
The following property types are available:
-
Boolean
-
Number (some code languages use Double and Int)
-
String
-
SemVer (
pre-release
andpatch
labels are not supported.) -
DateTime
Custom properties in code
Add a custom property to your connected app code for use in target groups or directly within feature flag configurations, then run the app to display the property options in the UI.
Custom properties are not limited to target groups; they can also be used individually inside a feature flag configuration. This allows for more granular flag targeting without requiring a predefined target group. For example, you can apply a custom property directly within flag rules to evaluate specific conditions based on user attributes.
For example, the following code within a connected JavaScript app results in the target group options displayed below.
Rox.setCustomStringProperty('company', getCompany()) Rox.setCustomBooleanProperty('isBetaUser', betaAccess()) Rox.setCustomBooleanProperty('isLoggedIn', isLoggedIn()) Rox.setCustomDateTimeProperty('isTargetDate', getTargetDate())
The DateTimeProperty is only available for CloudBees platform.
|
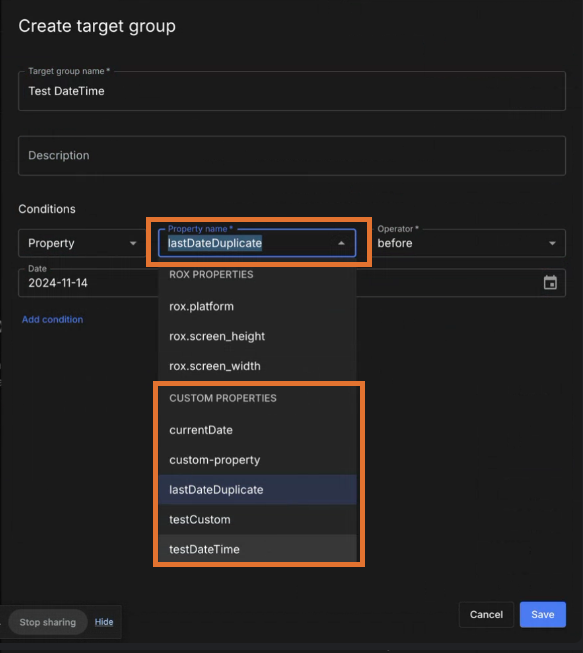
Usage examples: Explicit custom properties
Define explicit custom properties depending on the code language of your connected application:
Usage examples: Implicit custom properties
A dynamic custom property rule handler is called when an explicit custom property definition does not exist in the platform.
If you do not define this rule handler, the default function is activated, which tries to extract the property value from the context by its name.
A generic implementation of that handler is described by the following:
(propName, context) => context ? context[propName] : undefined
Create this handler for implicit custom properties, depending on the code language of your connected application:
If you are using a client-side SDK, and the value of a custom property changes while the app is running, such as after a user signs in, you may need to use Rox.unfreeze() .
|
Built-in targeting properties
The following table lists built-in targeting, in the format of rox.<attribute name>:
Attribute name | Description | Data type |
---|---|---|
|
Application release version. This is the value set in the SDK’s |
SemVer |
|
Universally unique identifier (UUID). |
String |
|
ISO 639 two-letter language code. For example, "en" for English, "es" for Spanish, and "zh" for Chinese. |
String |
|
Current time. |
DateTime |
|
Code language or framework name. For example, "Python" or "Gradle". |
String |
|
Screen height in pixels. |
Number |
|
Screen width in pixels. |
Number |
Use regular expressions
Regular expression (regEx) matching is an Operator option in target group creation.
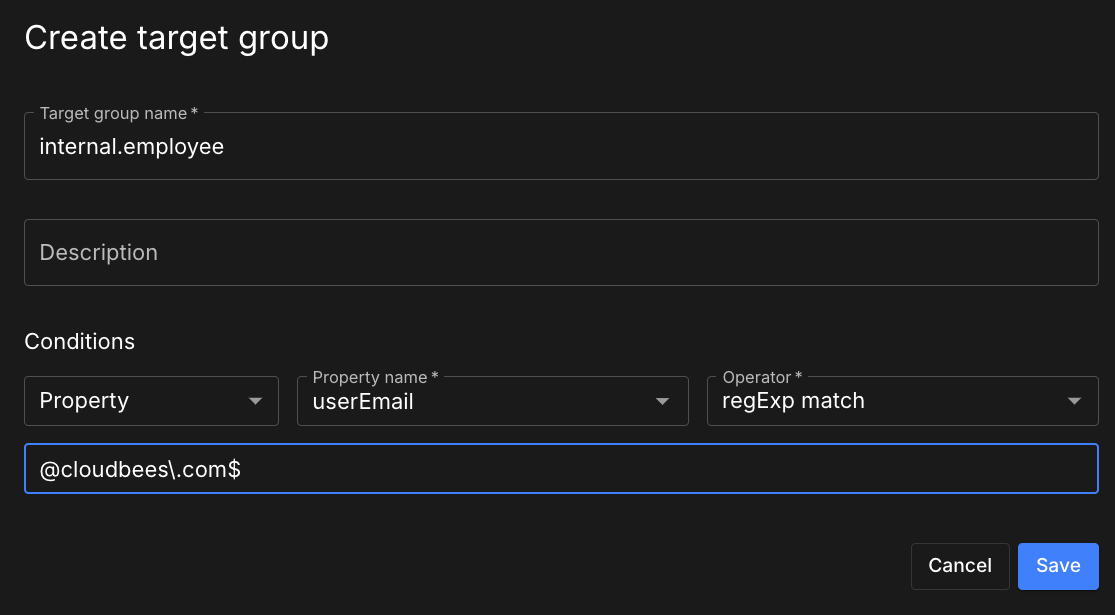
The underlying code on the SDK is:
import ( "regexp" ) boolean regexMatch(String str, String pattern){ matched, _ := regexp.MatchString(pattern, str) return matched; }
boolean regexMatch(String str, String pattern){ int options = 0; Pattern r = Pattern.compile(pattern, options); Matcher m = r.matcher(str); return m.find() }
function regexMatch(str, pattern) { var regex = new RegExp(pattern, ""); var match = regex.exec(str); return match ? true : false; }
function regexMatch(str, pattern) { var regex = new RegExp(pattern, ""); var match = regex.exec(str); return match ? true : false; }
bool regexMatch(String str, String pattern){ RegexOptions options = 0; Match match = Regex.Match(str, pattern, options); return match.Success; }
function regexMatch(str, pattern) { var regex = new RegExp(pattern, ""); var match = regex.exec(str); return match ? true : false; }
preg_match(pattern, src);
import re # In the above example # str is the email property value # pattern is "@cloudbees\.com$" re.search(pattern, src, 0)
function regexMatch(str, pattern) { var regex = new RegExp(pattern, ""); var match = regex.exec(str); return match ? true : false; }
# In the above example # str is the email property value # pattern is "@cloudbees\.com$" matched = !Regexp.new(pattern, 0).match(src).nil?
The following provides more information:
expect(parser.evaluateExpression('match("111", "222"')).toEqual(false); expect(parser.evaluateExpression('match("22222", ".*")')).toEqual(true); expect(parser.evaluateExpression('match("22222", "^2*$")')).toEqual(true); expect(parser.evaluateExpression('match("[email protected]", ".*(com|ca)")')).toEqual(true); expect(parser.evaluateExpression('match("[email protected]", ".*car\\.com$")')).toEqual(true); expect(parser.evaluateExpression('match("US", ".*IL|US")')).toEqual(true); expect(parser.evaluateExpression('match("US", "IL|US")')).toEqual(true); expect(parser.evaluateExpression('match("US", "(IL|US)")')).toEqual(true);
Manage custom properties in the UI
Create your own custom properties in the UI that can be used in target groups or as criteria for feature releases.
Access custom properties
To access custom properties, select
. Search for a specific custom property by entering all or part of a property name into Search.
Select ![]() ![]() |
Create a custom property
To create a custom property in the UI:
-
Select
. -
Select Create custom property.
-
Enter a Name.
-
(Optional) Enter a Description.
-
Select a data type from the options.
-
Select Save.
The custom property is created accordingly.
Update a custom property
You cannot update the custom property Name or Data type, but you can update the optional Description.
To update a custom property description:
-
Select
. -
Select
next to the property you want to update.
-
Select Edit property, and update or delete the description.
-
Select Update.
The custom property is updated accordingly.
Delete a custom property
A custom property cannot be deleted while it is still in use. You must first remove all references to it, such as conditions or configurations that use the property. Select Edit custom property to review and remove all references. Once all references are removed, the property can be safely deleted.
To delete a custom property:
-
Select
. -
Locate the custom property to be deleted.
-
Select
next to the property.
-
Select Delete. One of two dialogues displays.
-
The Delete (custom property) dialogue appears when the property is still in use. Follow these steps to remove the references:
-
Review the environments where the property is being used.
-
Verify that deletion is blocked; the Delete option is disabled until all references are removed.
-
Select the Edit flag configuration link to open the configuration interface and review how the property is applied in feature flag rules. Then, delete the configuration or condition as needed.
view the Delete (custom property) dialogue
Figure 3. Delete (custom property) dialogue -
To remove a full configuration, including all conditions within the configuration select Delete next to the configuration.
-
Select Save configuration to confirm changes.
Figure 4. Delete a configuration -
To remove conditions, select
next to the conditions you want to delete.
Figure 5. Delete a single condition -
Select Save configuration to confirm changes.
-
-
The safely delete (custom property) dialogue appears, indicating all references are removed.
Select Delete to permanently remove the custom property from the CloudBees platform.
Figure 6. Safely delete custom property dialogue
-
Once deleted, the custom property cannot be restored. |
Manage target groups in the UI
Create, update, and delete configured target groups for an application.
Access target groups
You can search in and sort the list of target groups.
To access target groups:
-
Select
. -
(Optional) Search for a specific target group, by entering all or part of a target group name into Search.
-
(Optional) Select an option in Sort by to sort the target group list by either ASCENDING or DESCENDING alphanumerical sort order.
The target groups are displayed according to your search or sort criteria.
Create a target group
Use custom properties or built-in attributes (ROX PROPERTIES) to define user segments. You can also create targeting dependent on other target groups.
You can only create nested statements based on target group options as long as there are no circular dependencies on other target groups. |
-
Select
. -
Select Create target group.
-
Enter a Name, an optional Description, and select one of the following conditions:
-
(Optional) Select the Property condition.
-
Select a Property name from the options.
-
Select an Operator from the options (including regular expression matching).
-
Enter a Value, if the property type is not a Boolean.
Select your keyboard Enter/Return key after entering each value to add multiple values when the equals one of operator is selected.
-
-
(Optional) Select the Target group condition.
-
Select a Matches … logical operator option.
-
Select a Target group from the options.
-
-
(Optional) Select Add condition to add more conditions.
-
Select one of the following logical operator options:
-
Matches all the following (AND operator)
-
Matches any of the following (OR operator)
All subsequent conditions use the same logical operator.
-
-
Configure either a Property or a Target group condition.
-
-
Select Save when all conditions are added.
Your target group is created, and listed in Target groups.
Select the next to a condition to remove it. However, you must have at least one condition. |
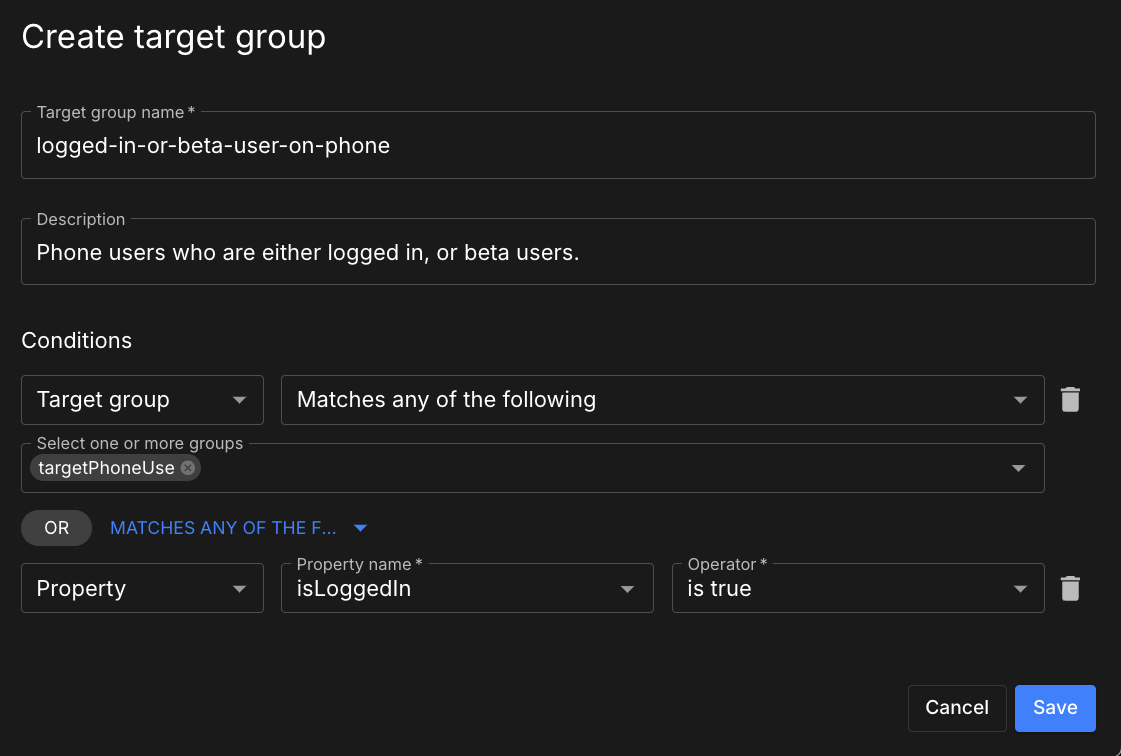
Update a target group
-
Select
. -
Select
next to the target group you want to delete.
-
Select Edit, and then make the desired updates.
-
Select Save.
The target group is updated accordingly.
Delete a target group
Delete any unused target group.
Before you can delete a target group, you must first remove all references to it in all flag configurations. |
A deleted target group is permanently removed from the application and all linked environments. Deletion is irreversible.
To delete a target group:
-
Select
. -
Select
next to the target group you want to delete.
-
Select Delete.
-
Remove all references to the target group in all flag configurations, if you have not already done so.
-
Select the
next to each displayed environment.
-
For each flag in each environment, select Edit configuration.
Figure 8. Select Edit configuration to remove the target group reference. -
Remove the target group reference.
-
Return to the target group you want to delete and select Delete.
-
-
Select Delete.
The selected target group is deleted and removed from the target group list.